If you've been around the internet recently, you've probably heard about the boom in artificial intelligence and chatbots.
And that's why I got to jump on the bandwagon as well
But as a programming enthusiast, I've always wondered how exactly AI algorithms work, and especially how AI can learn to create art, finish my math homework and even write code!
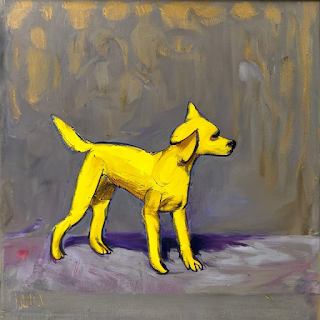%20(1).png) |
Created by the "Stable Diffusion" AI |
It turns out there is LOTS of stuff going on under the hood. There are dozens of algorithms and systems, some just basic machine learning, others entire neural networks to simulate brains. Luckily though, there are many libraries and APIs that can help you out with the more complex stuff.
My first attempts
When I was first starting with AI, I decided to choose JavaScript as my language, mostly because I was most comfortable with it and knew quite a bit already. I went with the
BrainJS library. It's quite simple to use and you do not have to have much knowledge of neural networks to use it. I wanted to create generative AI at first, so I started writing LOTS of training data for the AI model. Training data is a must for many AIs. A new AI has no information about anything, so what we need to do is feed it training data. Say you want to create a story telling AI, before the AI understands how to write stories you will have to give it lots of story examples, in order to give it "ideas". In BrainJS, we can do this by giving it lots of data in JSON like format.
I ended up creating several projects with this library, but I soon realized that it was going to take a lot of time and effort to make generative AI as advanced as I'd like. Along with that, it was going to take a lot of computing power to train large models, something my little laptop would not be strong at. In addition, I found that JavaScript is not the ideal language to develop AI in practically.
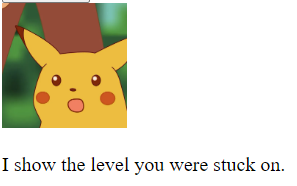 |
My AI meme maker (JS) |
Luckily though, many APIs were becoming available and I decided to give some a try.
AI with ChatBot APIs
APIs are a good option, you don't have to code the entire AI, by borrowing code from other AI services, while still being able to customize it to your needs. Think of APIs like ordering something off Amazon, you order the item you'd like and they will deliver it to you, but in this case we are ordering AI chatbots.
It was a daunting task to find the API I wanted, but I decided to go with this one called
HuggingChat, a chatbot, similar to ones you may have heard of like, ChatGPT and Bard. It is free, open source and has a Python API.
In order to use the API you must use the Python Module associated with it:
pip install hugchat
Setting up the module is pretty simple, first import the necessary packages:
from hugchat import hugchat
from hugchat.login import Login
In order to use the API, you need a HuggingFace account. You can make one for free at their website, https://huggingface.co/
You will need this account, because you will have to insert your account details into your code in order for it to work:
sign = Login("Put your email in here", "and your password here")
cookies = sign.login()
sign.saveCookies()
Finally, setup the chatbot by using this code snippet:
chatbot = hugchat.ChatBot(cookies=cookies.get_dict())
Now it should be working, to test it try something like this:
print(chatbot.chat("Hello"))
Now, if the code and API is working correctly, you'll get a response in your shell that looks like this:
Hello! I am your AI Assistant, ready to assist you. How can I help?
Pretty interesting! Now that you've got the base code ready, you might want to add some other things.
For my first project I decided to make a bot that is a little funny. I ended up using this prompt for it:
Answer this question, except this time, provide an incorrect, funny, sarcastic answer. Here is the question:
As you can see I got some funny responses 😆
This may look daunting, but it's actually just the prompt- and a few lines of code:
messages = input()
print(chatbot.chat("Answer this question, except this time, provide an incorrect, funny, sarcastic answer. Here is the question: " + messages))
Plus you can use chatbot.chat anywhere, so you can do even more things than just make chatbots. For example, I've been working on an AI app that can help you edit documents and more. It connects to your text document and then uses chatbot.chat to give suggestions. (Maybe I'll release it soon 😉)
Hopefully you found this as interesting as I did and I'd love to see what you create!
Also- You may want to check out the full documentation on the Python API - https://github.com/Soulter/hugging-chat-api
There are more options and settings there that I did not cover. I'd like to thank the people that made this API as it is extremely helpful and I'm glad to see more free options coming.